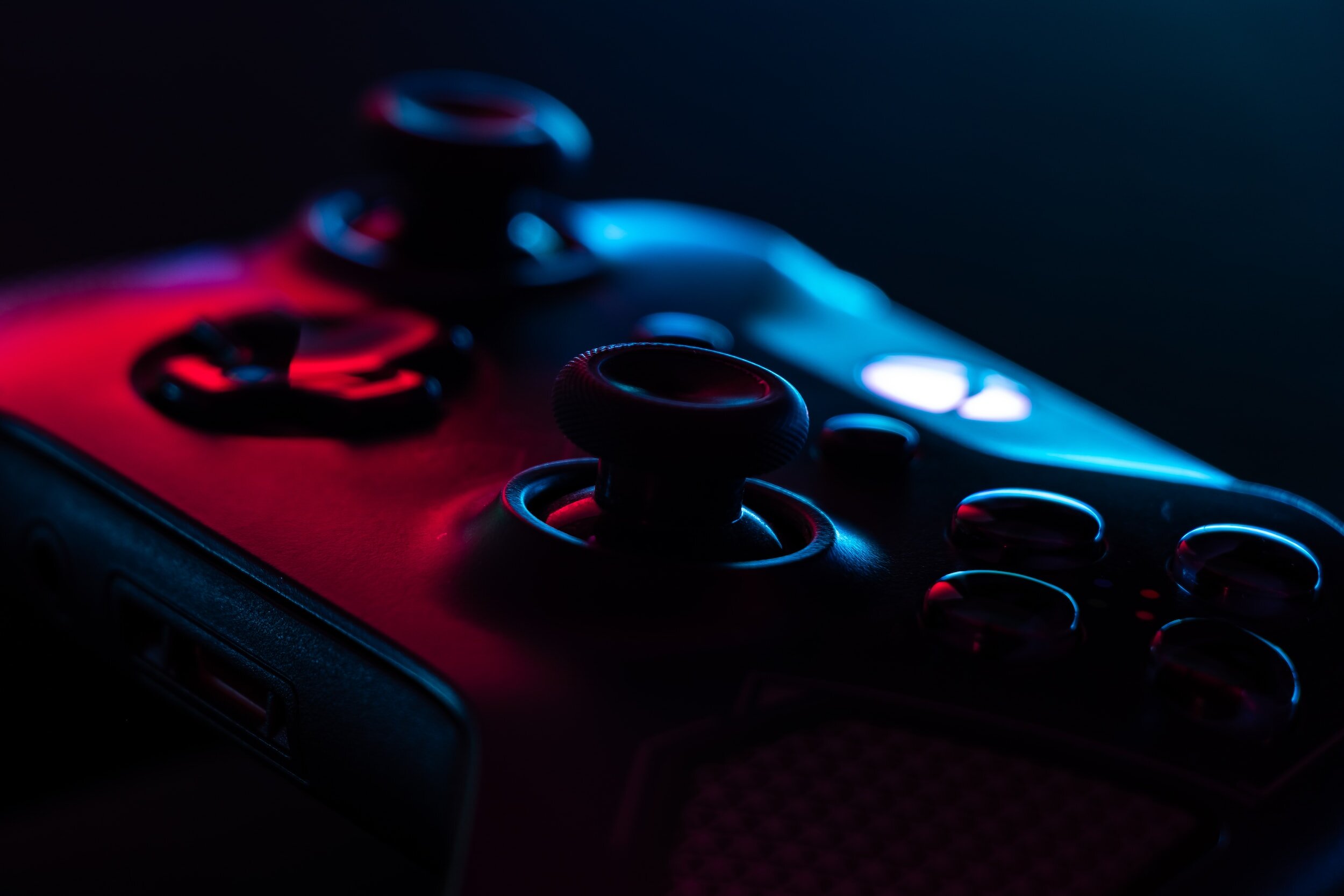
Joystick C++ library v2.0.1
Cross-platform (Windows and Linux) lightweight C++ library to capture joysticks/gamepads input
Overview
Joystick is a C++ library which assists developers in seamlessly interfacing with various types of joysticks, all without relying on third-party dependencies. This library offers an array of methods designed for effortless reading from buttons, axes, and hat inputs. Its intuitive design ensures simplicity and ease of use, allowing developers to work efficiently without unnecessary complexity and 3rd party dependencies. The library has simple interface:
class Joystick { public: /// find joysticks. std::vector<JoystickInfo> findJoysticks(); /// Method to open a joystick. bool openJoystick(int id); /// Close joystick. void closeJoystick(); /// Check status of a button. bool getButtonState(int buttonId); /// Get hat value. int getHatValue(); /// Get axis value. int getAxisValue(int axisId); };
How to use
Source code bellow is simple application which uses Joystick library to get button states:
#include <iostream> #include <thread> #include <chrono> #include "Joystick.h" int main(void) { // Create joystick controller. cr::utils::Joystick joyStickController; // Read number of connected joysticks. std::vector<cr::utils::JoystickInfo> joysticks; joysticks = joyStickController.findJoysticks(); // Open first joystick joyStickController.openJoystick(joysticks.at(0).id); // Print joystick infos. std::cout << "Joystick name : " << joysticks.at(0).name << std::endl; std::cout << "Number of button : " << joysticks.at(0).numButtons << std::endl; std::cout << "Number of axes : " << joysticks.at(0).numAxes << std::endl; // Read all avaliable buttons one by one while (true) { for (int i = 0; i < joysticks.at(0).numButtons; ++i) { if (joyStickController.getButtonState(i) == true) std::cout << "Button : " << i << " pressed" << std::endl; } std::this_thread::sleep_for(std::chrono::milliseconds(100)); } return 0; }
Price and Terms
The Joystick C++ library is supplied under a license in source code. Library pricing is available upon request (info@constantrobotics.com).
Downloads
Joystick C++ library programmer’s manual: DOWNLOAD