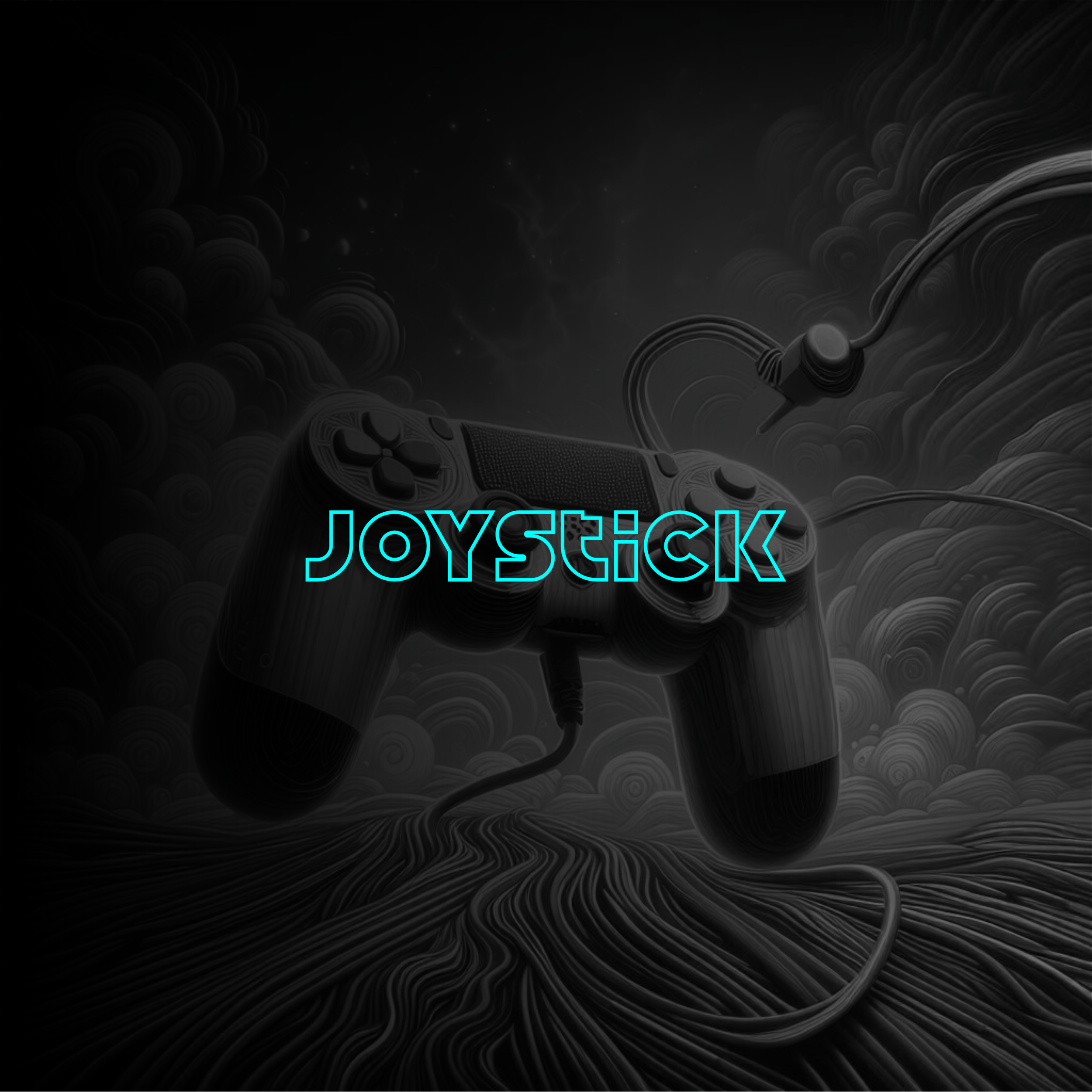
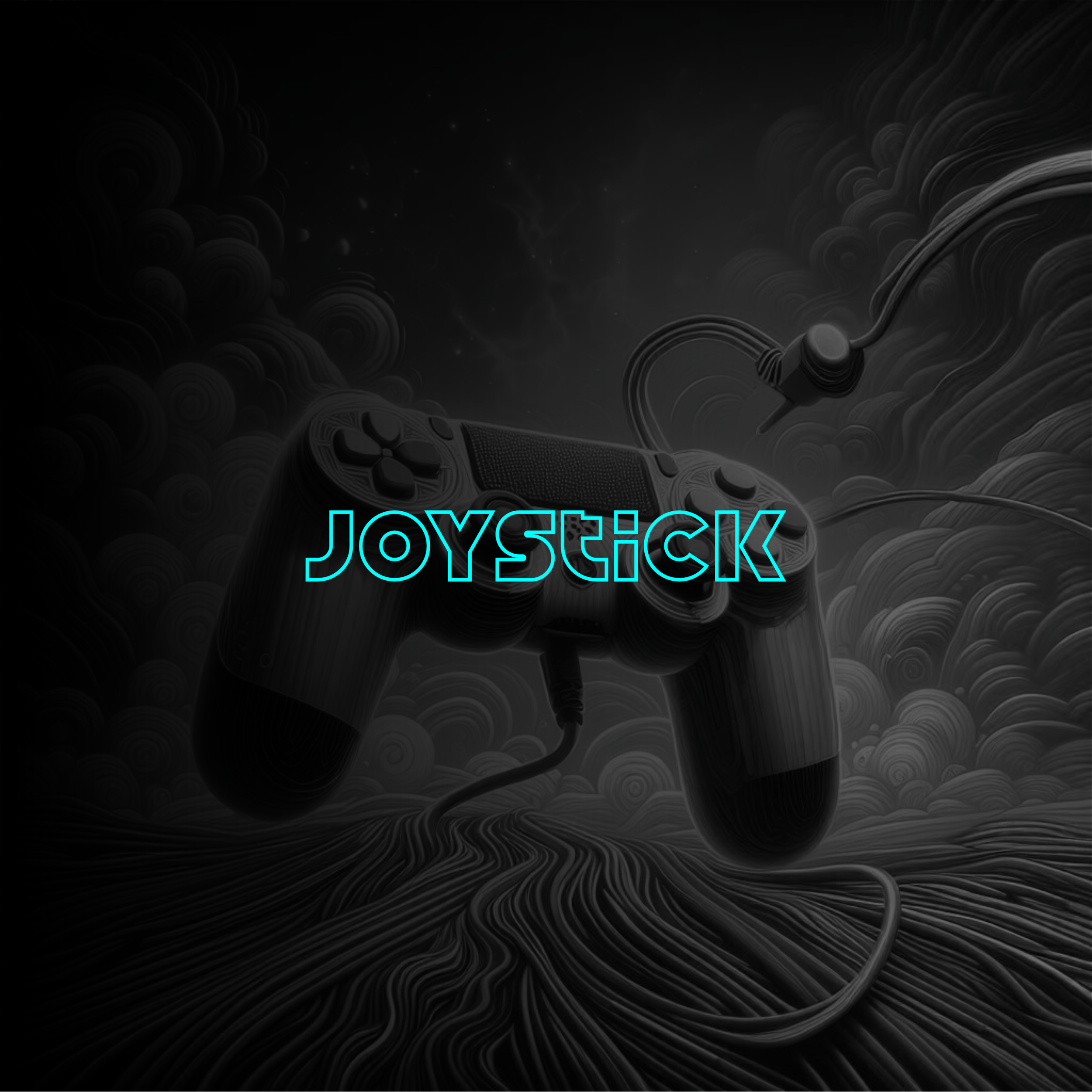
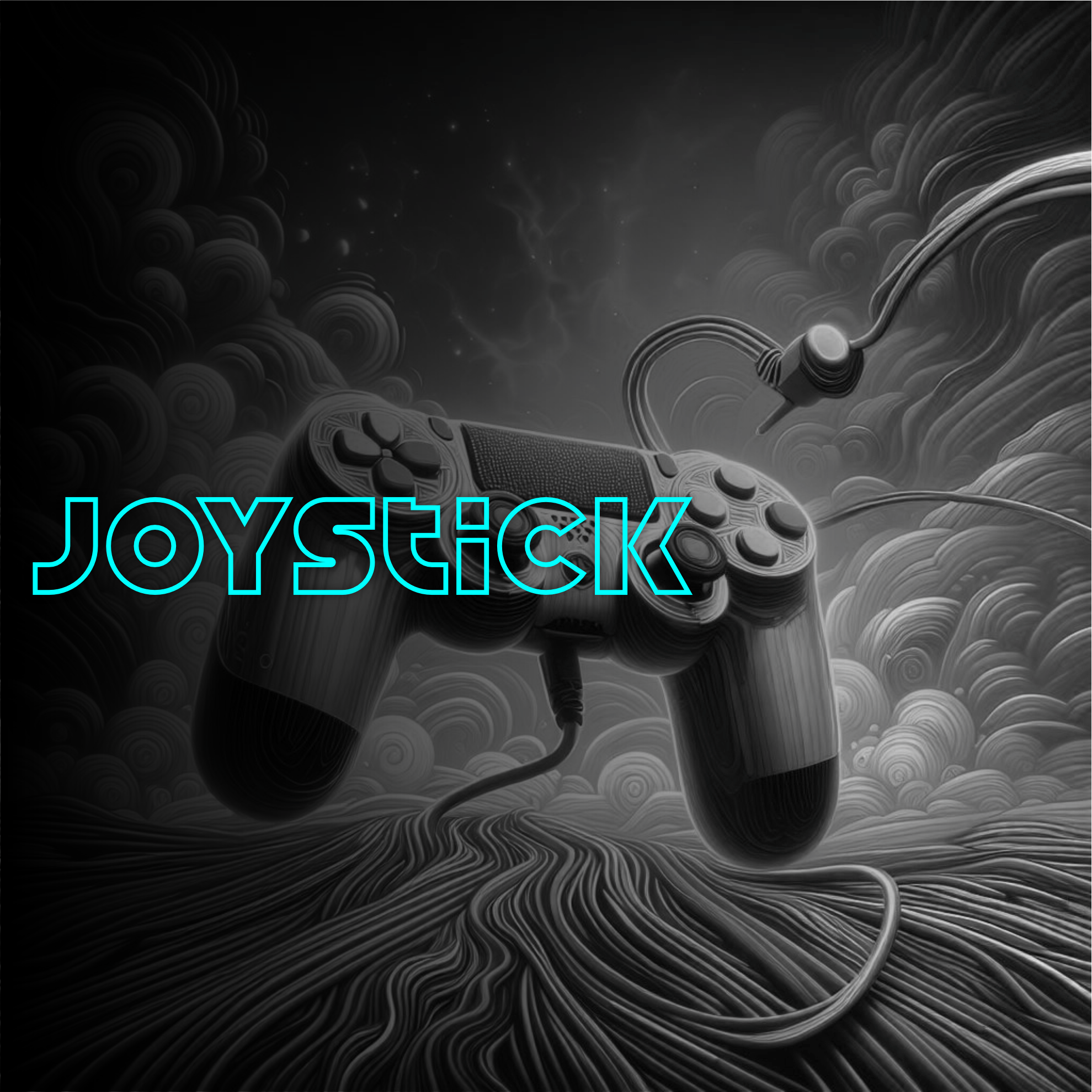
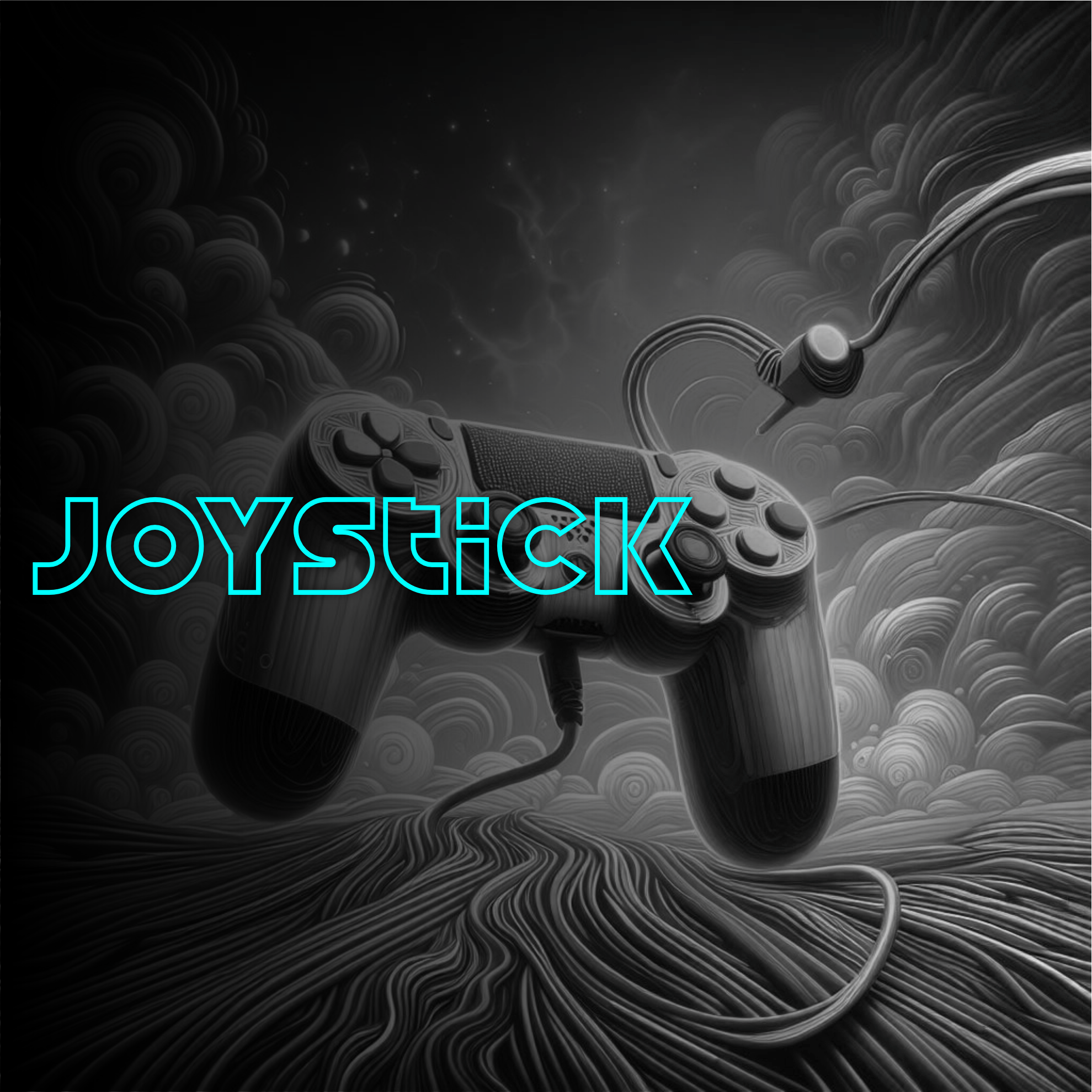
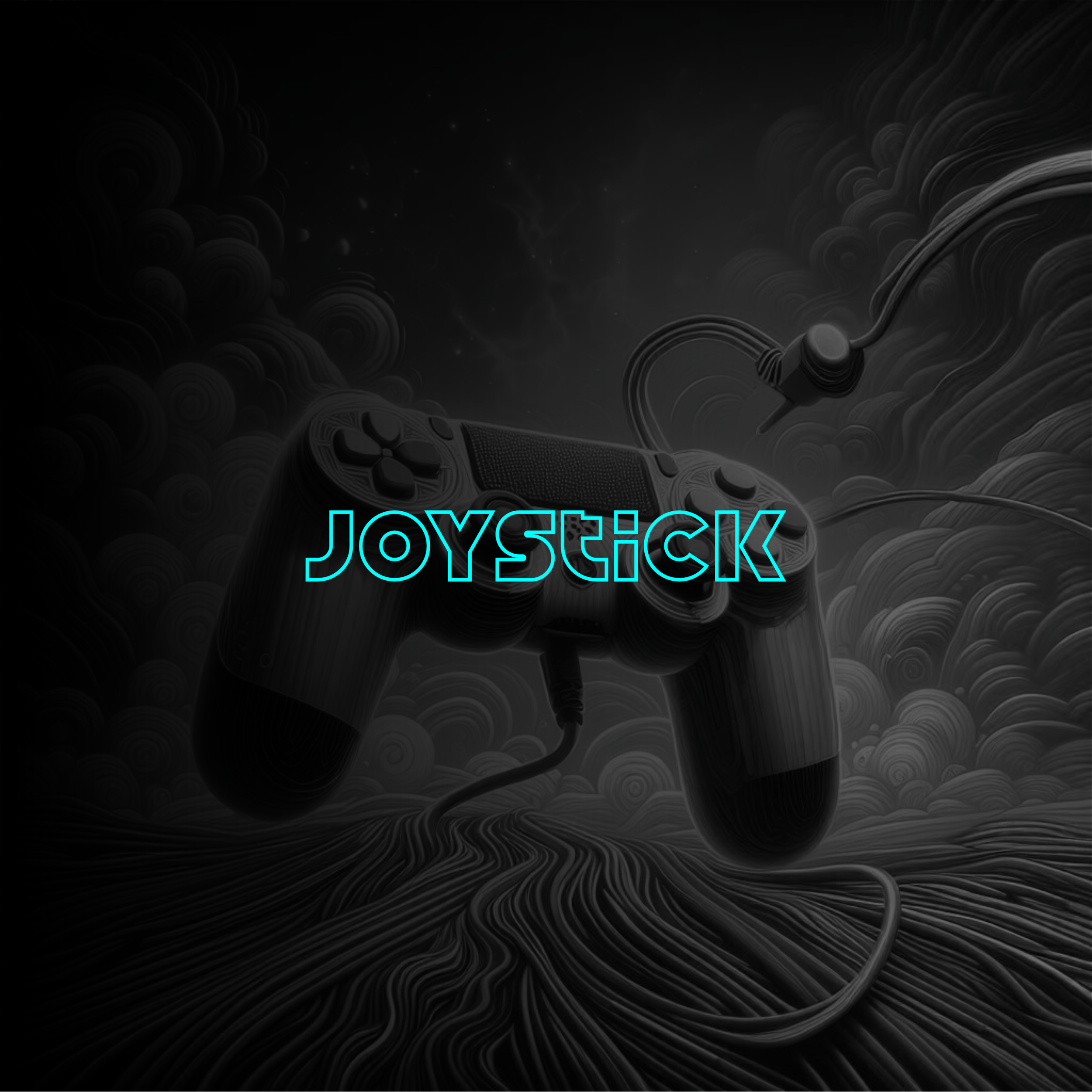
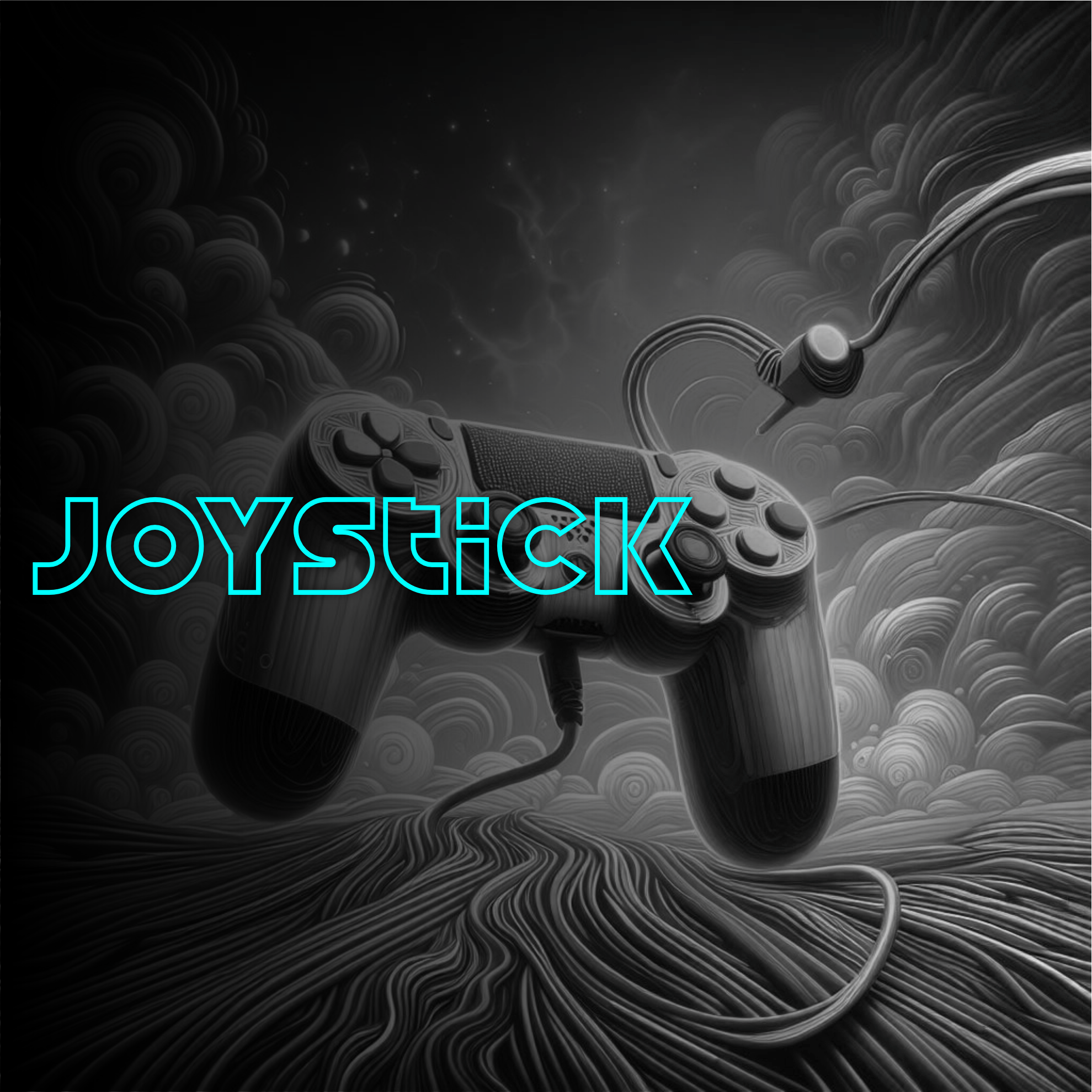
Joystick C++ lib. Simple cross-platform library to work with joysticks
Joystick v2.0.5 is a C++ library which assists developers in seamlessly interfacing with various types of joysticks, all without relying on third-party dependencies.
We sell source code of this library as is, without future updates and technical support according to perpetual non-exclusive license. You pay once and can use this library in your software and hardware products without limits. Please read the license agreement before purchasing: LICENSE. You can buy technical support service for this product.
Joystick v2.0.5 is a C++ library which assists developers in seamlessly interfacing with various types of joysticks, all without relying on third-party dependencies.
We sell source code of this library as is, without future updates and technical support according to perpetual non-exclusive license. You pay once and can use this library in your software and hardware products without limits. Please read the license agreement before purchasing: LICENSE. You can buy technical support service for this product.
Joystick v2.0.5 is a C++ library which assists developers in seamlessly interfacing with various types of joysticks, all without relying on third-party dependencies.
We sell source code of this library as is, without future updates and technical support according to perpetual non-exclusive license. You pay once and can use this library in your software and hardware products without limits. Please read the license agreement before purchasing: LICENSE. You can buy technical support service for this product.
Purchase options
You can buy the software by bank transfer. Bank transfer available only for companies. To buy software by bank transfer please send us request to info@constantrobotics.com. Also, you can buy technical support service for this product.
Overview
Joystick is a C++ library which assists developers in interfacing with various types of joysticks, all without relying on third-party dependencies. The library is compatible with Linux (uses Linux native joysticks interface) and Windows (uses WinAPI). The library doesn't have third party dependencies. The library is a CMake project and utilizes C++17 standard.
Downloads
Programmer’s manual: DOWNLOAD
Simple interface
namespace cr
{
namespace utils
{
/// Joystick class.
class Joystick
{
public:
/// Get Joystick class version.
static std::string getVersion();
/// Class constructor.
Joystick();
/// Class destructor.
~Joystick();
/// Find available joysticks.
std::vector<JoystickInfo> findJoysticks();
/// Open joystick.
bool openJoystick(int id);
/// Close joystick.
void closeJoystick();
/// Check status of a button.
bool getButtonState(int buttonId);
/// Get hat value.
int getHatValue();
/// Get axis value.
int getAxisValue(int axisId);
};
}
}
Simple example
#include <iostream>
#include <thread>
#include <chrono>
#include "Joystick.h"
int main(void)
{
// Create joystick controller.
cr::utils::Joystick joyStickController;
// Read number of connected joysticks.
std::vector<cr::utils::JoystickInfo> joysticks;
joysticks = joyStickController.findJoysticks();
// Open first joystick
joyStickController.openJoystick(joysticks.at(0).id);
// Print joystick infos.
std::cout << "Joystick name : " << joysticks.at(0).name << std::endl;
std::cout << "Number of button : " << joysticks.at(0).numButtons << std::endl;
std::cout << "Number of axes : " << joysticks.at(0).numAxes << std::endl;
// Read all avaliable buttons one by one
while (true)
{
for (int i = 0; i < joysticks.at(0).numButtons; ++i)
{
if (joyStickController.getButtonState(i) == true)
std::cout << "Button : " << i << " pressed" << std::endl;
}
std::this_thread::sleep_for(std::chrono::milliseconds(100));
}
return 0;
}