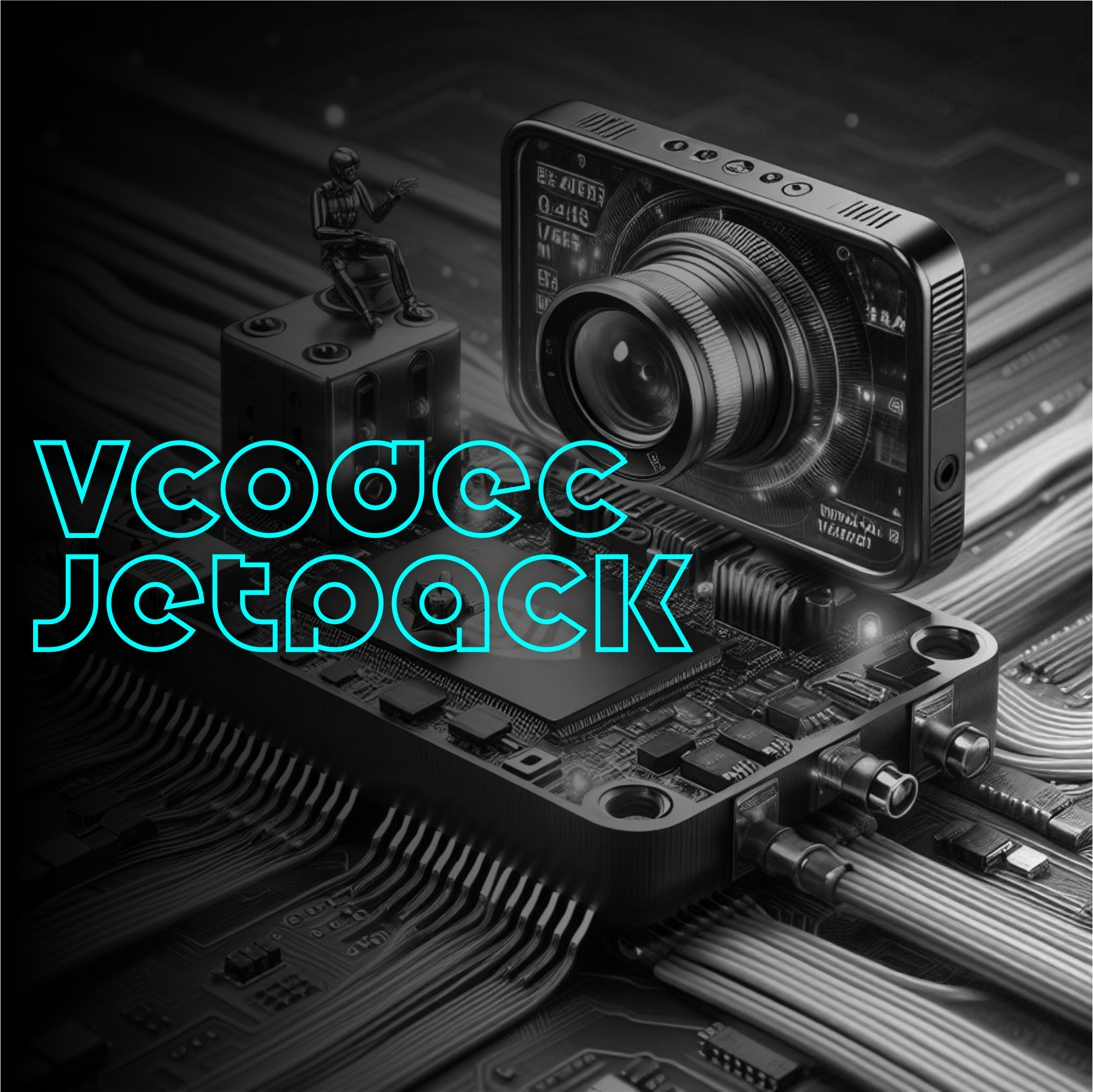
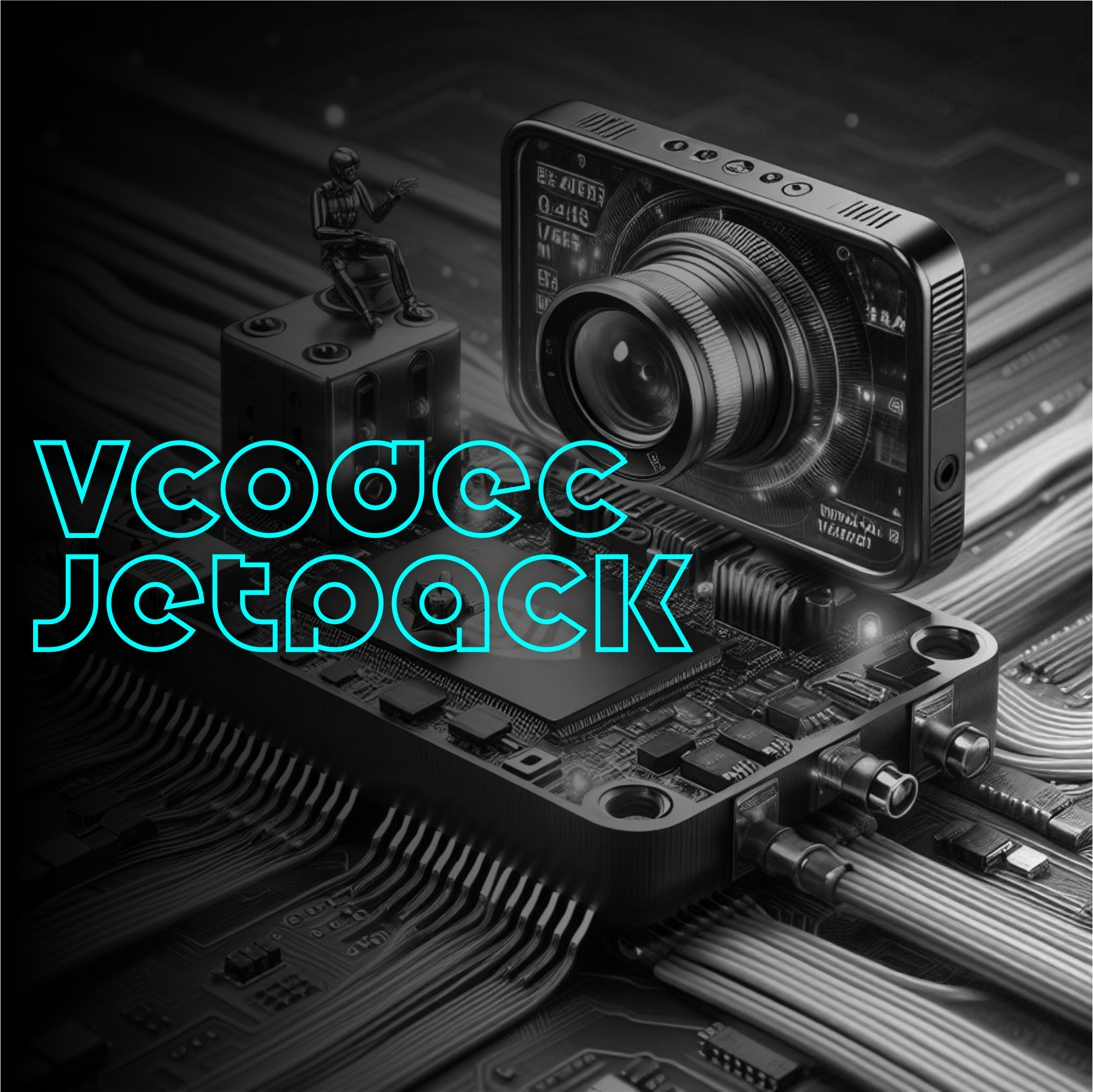
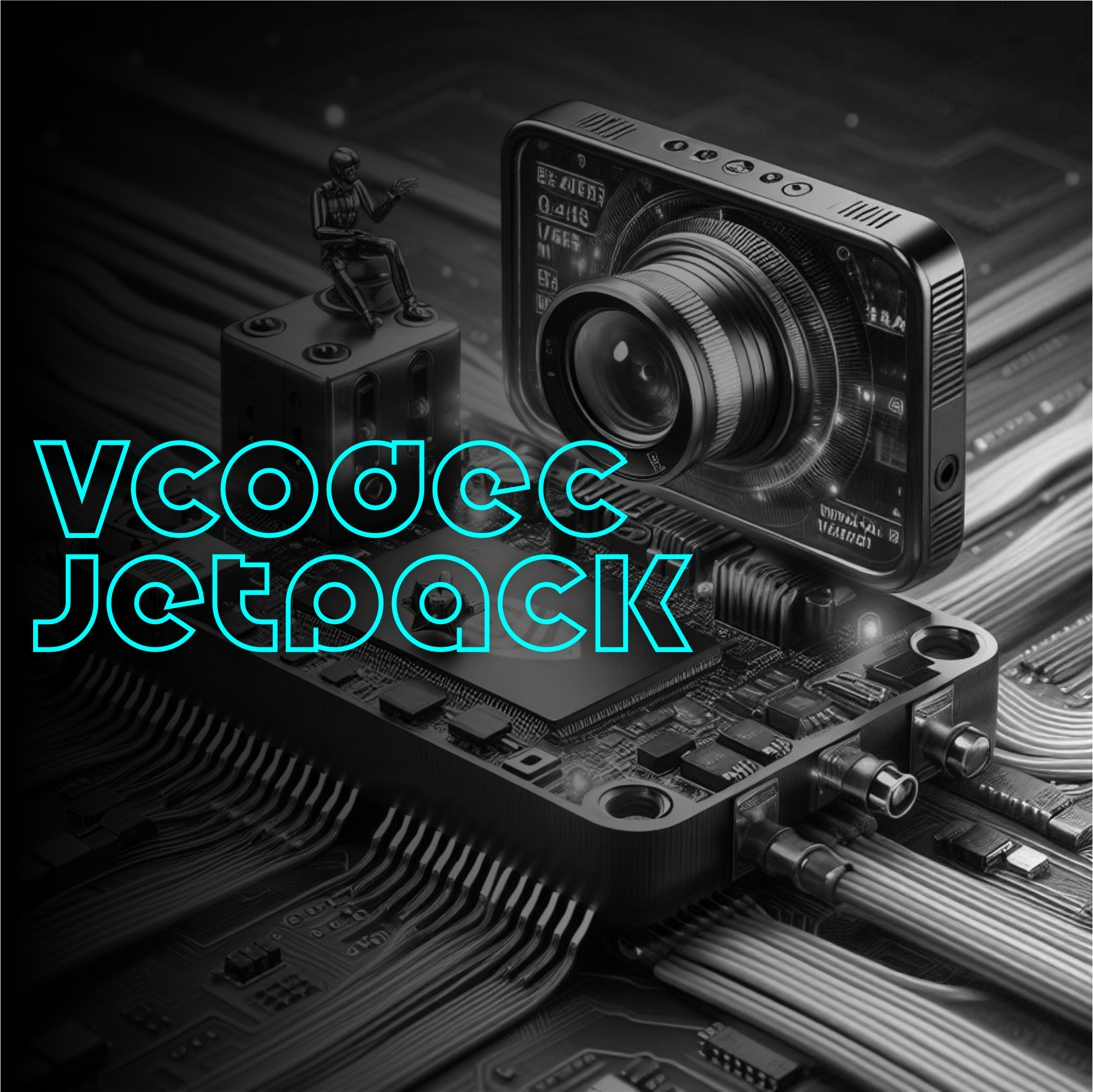
VCodecJetPack C++ lib. Fast video codec (H264, HEVC) for Nvidia Jetson platforms
VCodecJetPack C++ library provides hardware video encoding for H264 and HEVC codecs for Jetson platforms based on Jetson Multimedia API.
LICENSE: We sell source code of this library as is, without future updates and technical support according to perpetual non-exclusive royalty-free license. You pay once and can use this library in your software and hardware products without limits. Please read the license agreement before purchasing: DOWNLOAD LICENSE. You can buy technical support service for this product.
VCodecJetPack C++ library provides hardware video encoding for H264 and HEVC codecs for Jetson platforms based on Jetson Multimedia API.
LICENSE: We sell source code of this library as is, without future updates and technical support according to perpetual non-exclusive royalty-free license. You pay once and can use this library in your software and hardware products without limits. Please read the license agreement before purchasing: DOWNLOAD LICENSE. You can buy technical support service for this product.
VCodecJetPack C++ library provides hardware video encoding for H264 and HEVC codecs for Jetson platforms based on Jetson Multimedia API.
LICENSE: We sell source code of this library as is, without future updates and technical support according to perpetual non-exclusive royalty-free license. You pay once and can use this library in your software and hardware products without limits. Please read the license agreement before purchasing: DOWNLOAD LICENSE. You can buy technical support service for this product.
Purchase options
You can by this software online by card or you can buy the software by bank transfer. Bank transfer available only for companies. To buy software by bank transfer please send us request to info@constantrobotics.com. Also, you can buy technical support service for this product.
Downloads
Programmer’s manual: DOWNLOAD
Overview
VCodecJetPack C++ library provides hardware video encoding for H264 and HEVC codecs for Jetson platforms based on Jetson Multimedia API. VCodecJetPack class inherits interface and data structures from open source VCodec library and also includes Logger open source library which provides function for print logs. VCodecJetPack uses Jetson Jetson Multimedia API. The library provides simple programming interface to be implemented in different C++ projects. The library was written with C++17 standard. The libraries are supplied as source code only. The library is a CMake project. Encoding time on Jetson Orin NX:
H264 codec, 2560x1440 - 14.4 msec, 1920x1080 - 8.6 msec, 1280x720 - 4.2 msec, 640x512 - 2 msec.
HEVC codec, 2560x1440 - 13.8 msec, 1920x1080 - 8.4 msec, 1280x720 - 2.3 msec, 640x512 - 2 msec.
Simple interface
class VCodecJetPack : public VCodec
{
public:
/// Get library version.
static std::string getVersion();
/// Set parameter value.
bool setParam(VCodecParam id, float value) override;
/// Get parameter value.
float getParam(VCodecParam id) override;
/// Encode video frame.
bool transcode(Frame& src, Frame& dst) override;
/// Execute command.
bool executeCommand(VCodecCommand id) override;
};
Simple example
#include <iostream>
#include "VCodecJetPack.h"
int main(void)
{
// Create codec.
cr::video::VCodec* videoCodec = new cr::video::VCodecJetPack();
// Set codec parameters.
videoCodec->setParam(cr::video::VCodecParam::BITRATE_KBPS, 7500);
videoCodec->setParam(cr::video::VCodecParam::GOP, 30);
videoCodec->setParam(cr::video::VCodecParam::FPS, 30);
// Create NV12 frame.
const int width = 1280;
const int height = 720;
cr::video::Frame frameNv12(width, height, cr::video::Fourcc::NV12);
// Fill NV12 frame by random values.
for (uint32_t i = 0; i < frameNv12.size; ++i)
frameNv12.data[i] = (uint8_t)i;
// Create output HEVC frame.
cr::video::Frame frameHEVC(width, height, cr::video::Fourcc::HEVC);
// Create output file.
FILE *outputFile = fopen("out.hevc", "w+b");
// Params for moving object.
int objectWidth = 128;
int objectHeight = 128;
int directionX = 1;
int directionY = 1;
int objectX = width / 4;
int objectY = height / 2;
// Encode and record 200 frames.
for (uint32_t n = 0; n < 200; ++n)
{
// Draw moving object.
memset(frameNv12.data, 128, width * height);
for (int y = objectY; y < objectY + objectHeight; ++y)
for (int x = objectX; x < objectX + objectHeight; ++x)
frameNv12.data[y * width + x] = 255;
objectX += directionX;
objectY += directionY;
if (objectX >= width - objectWidth - 5 || objectX <= objectWidth + 5)
directionX = -directionX;
if (objectY >= height - objectHeight - 5 || objectY <= objectHeight + 5)
directionY = -directionY;
// Encode.
if (!videoCodec->transcode(frameNv12, frameHEVC))
{
std::cout << "Can't encode frame" << std::endl;
continue;
}
// Write to file.
fwrite(frameHEVC.data, frameHEVC.size, 1, outputFile);
}
// Close file.
fclose(outputFile);
return 1;
}